Buy Now Button Animation With HTML CSS JavaScript
Welcome to our tutorial on how to create a buy now button animation using HTML, CSS, and JavaScript. In today’s fast-paced online shopping world, it’s important to have a clear and attention-grabbing call to action for your customers. A well-designed buy now button can make all the difference in converting website visitors into paying customers.
In this tutorial, we will guide you through the process of creating a sleek and interactive buy now button animation using the power of HTML, CSS, and JavaScript. Whether you are a seasoned web developer or just starting out, this tutorial will provide you with the skills and knowledge you need to add a professional and dynamic buy now button to your website. So let’s get started!
For additional HTML, CSS, and JavaScript projects, see our website.
Source Code:
HTML:
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="UTF-8">
<title>Buy Now Button Animation</title>
<!-- Page Essentials Link e Meta tags -->
<title>Buy Now Button Animation</title>
<meta http-equiv="Content-Type" content="text/html;charset=UTF-8"/>
<meta http-equiv="X-UA-Compatible" content="IE=Edge,chrome=1" />
<meta name="viewport" content="width=device-width, minimum-scale=1.0, maximum-scale=1.0, user-scalable=no">
<meta name="theme-color" content="#4c4c4c">
<!-- Css -->
<link href="https://fonts.googleapis.com/css?family=Roboto+Condensed" rel="stylesheet">
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.7.2/css/all.css" integrity="sha384-fnmOCqbTlWIlj8LyTjo7mOUStjsKC4pOpQbqyi7RrhN7udi9RwhKkMHpvLbHG9Sr" crossorigin="anonymous">
<meta name="viewport" content="width=device-width, initial-scale=1"><link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/meyer-reset/2.0/reset.min.css">
<link rel="stylesheet" href="./style.css">
</head>
<body>
<!-- partial:index.partial.html -->
<div class="balance">
<i class="fas fa-coins"></i>
<span></span>
</div>
<div class="buy">
<i class="fa fa-check" aria-hidden="true"></i>
<span>Buy Now!</span>
<small>US$39,99</small>
<div class="download">
<i class="fas fa-shipping-fast" aria-hidden="true"></i>
</div>
</div>
<!-- partial -->
<script src='https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js'>
</script><script src="./script.js"></script>
</body>
</html>
——————————
📂 Important Links:
——————————
>> Learn Graphics Design & Make A Successful Profession.
>> Canva Makes Graphics Design Easy.
>> Start Freelancing Today & Earn Money.
>> Make Video Editing As Your Profession.
CSS:
*, *:before, *:after {
margin: 0;
padding: 0;
outline: none;
-ms-box-sizing: border-box;
-o-box-sizing: border-box;
box-sizing: border-box;
font-size:100%;
vertical-align:baseline;
background:transparent;
transition: all .2s ease;
}
html, body {
height: 100%;
width: 100%;
background-color: #e0e0e0;
font-family: sans-serif;
overflow: hidden;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
zoom: 1.05;
}
html, body, .buy, .download {
display: -moz-flex;
display: -ms-flex;
display: -o-flex;
display: flex;
-moz-justify-content: center;
-ms-justify-content: center;
-o-justify-content: center;
justify-content: center;
-moz-align-items: center;
-ms-align-items: center;
-o-align-items: center;
align-items: center;
font-family: 'Roboto Condensed', sans-serif;
}
.buy {
color: #4c4c4c;
border: 2px solid #4c4c4c;
text-align: center;
text-transform: uppercase;
font-weight: bold;
width: 190px;
height: 45px;
font-size: 15px;
cursor: pointer;
position: relative;
border-radius: 5px;
letter-spacing: 1.3px;
-ms-box-shadow: 0 10px 20px rgba(0, 0, 0, 0.05), 0 6px 6px rgba(0, 0, 0, 0.04);
-o-box-shadow: 0 10px 20px rgba(0, 0, 0, 0.05), 0 6px 6px rgba(0, 0, 0, 0.04);
box-shadow: 0 10px 20px rgba(0, 0, 0, 0.05), 0 6px 6px rgba(0, 0, 0, 0.04);
transition: all .2s linear;
}
.buy small {
font-weight: 100;
font-size: 14px;
margin-left: 5px;
opacity: .9;
}
.fa-check, .fa-shipping-fast {
display: none;
font-size: 17px;
}
.download {
position: absolute;
left: 50%;
top: 50%;
transform: translate(-50%, -50%);
width: 50px;
height: 100%;
border-radius: 50%;
background-color: #21d49a;
color: #F5F5F5;
transition: all .1s ease;
opacity: 0;
}
.download, .loading:before {
-webkit-opacity: 0;
-moz-opacity: 0;
-ms-opacity: 0;
-o-opacity: 0;
opacity: 0;
visibility: hidden;
}
.download.active {
border-radius: 0;
width: 100%;
will-change: opacity, visibility, width, height;
}
.download.active:after {
content: 'Track Package';
position: absolute;
display: block;
margin-left: 20px;
white-space: nowrap;
font-size: 16px;
color: #f5f5f5;
}
.download.active, .processing:before {
-webkit-opacity: 1;
-moz-opacity: 1;
-ms-opacity: 1;
-o-opacity: 1;
opacity: 1;
visibility: visible;
}
.fa-shipping-fast {
margin-right: auto;
margin-left: 18px;
font-size: 14px;
}
.success {
color: #21d49a;
border-color: #21d49a;
will-change: color, border-color;
}
.loading {
cursor: default;
height: 10px;
color: transparent;
will-change: cursor, height, color;
}
.loading:before {
content: 'Processing Payment...';
position: absolute;
top: 0;
left: 50%;
display: block;
margin: 0 auto;
white-space: nowrap;
font-size: 11.5px;
color: #4c4c4c;
transform: translateX(-50%);
will-change: transform;
}
.processing:before {
top: -20px;
}
.loading:after {
content: '';
position: absolute;
bottom: 0;
left: 0;
width: 0%;
height: 100%;
background-color: #4c4c4c;
-webkit-animation: loading 2s ease .75s 1 forwards;
animation: loading 2s ease .75s 1 forwards;
will-change: width;
}
@-webkit-keyframes loading {
0% {
width: 0%;
}
70% {
width: 50%;
}
100% {
width: 100%;
}
}
@keyframes loading {
0% {
width: 0%;
}
70% {
width: 50%;
}
100% {
width: 100%;
}
}
.balance {
position: fixed;
top: 10%;
right: 5%
}
.balance span {
margin-left: 5px;
}
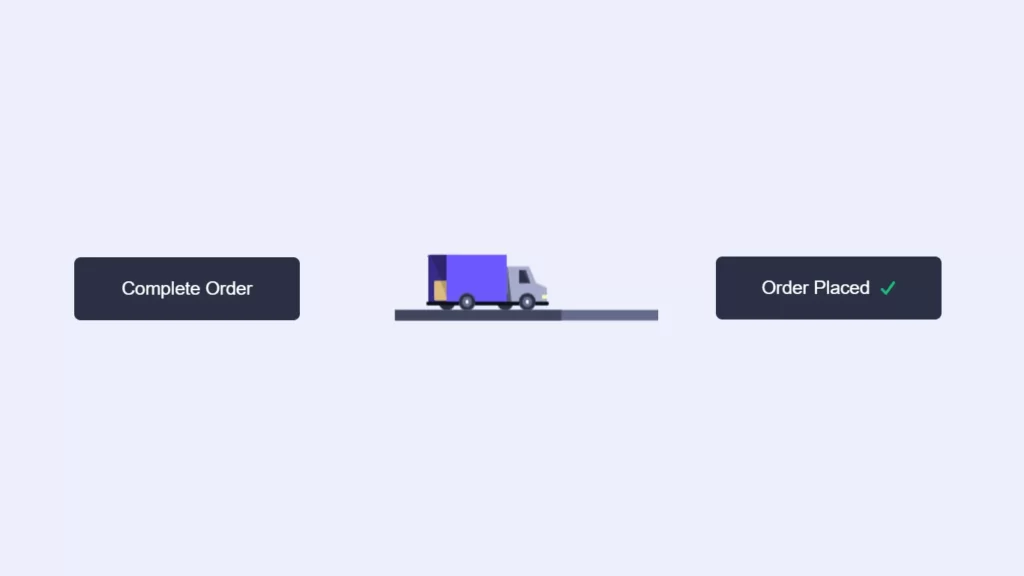
JavaScript
$(document).ready(function() {
var canDownload = true;
var money = 100;
$('.balance span').text(money);
$('.buy').on('click', function() {
if(canDownload === true) {
$(this).addClass('loading').find('span, small').hide();
setTimeout(function() {
$('.loading').addClass('processing');
canDownload = false;
setTimeout(function() {
$('.buy').removeClass('processing');
setTimeout(function() {
$('.buy').removeClass('loading').addClass('success').find('.fa-check').fadeIn(100);
setTimeout(function() {
money -= 39.99;
$('.balance span').text(money);
$('.download').addClass('active').children().fadeIn(100, function() {
$('meta[name="theme-color"]').attr('content','#21d49a');
$('.buy').css('background-color','#21d49a');
});
}, 1000);
}, 400);
}, 2800);
}, 300);
}
});
});