Build A Clock With JavaScript | JavaScript Project
Lets Build a Clock using javascript. welcome to our another javascript projects. as animations, so we’re gonna create a little mini series over this, probably a two part series where we’re going to cover everything about this library that I can possibly you think so. This article is not owned the overview, but we are going to get this working in some actual code.
Source Code:
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>Digital Clock</title>
<link rel="stylesheet" href="clock2.css">
</head>
<body>
<div id="clock">8:10:45</div>
<script src="clock2.js"></script>
</body>
</html>
CSS:
#clock {
font-size: 175px;
width: 900px;
margin: 200px;
text-align: center;
border: 2px solid black;
border-radius: 20px;
}
JavaScript
Code in JavaScript: Follow the instructions listed below for JavaScript.
- First, create a function called “showTime.”
- Step 2 is to create a Date object instance.
- Step 3: Using the Date object’s methods, obtain “hours,” “minutes,” and “seconds.”
- Set AM/PM based on the hour value in step four. Since the Date object uses a 24-hour format, we reset the hour to 1 when it exceeds 12. Additionally, the AM/PM shifts in line with it.
- Step 5: Create a string with the same HH:MM:SS formatting, replacing the values for the hour, minute, and second with those obtained from Date object methods.
- Step 6: Using the innerHTML property, now change the string variable in the “div.”
- Step 7: Use the setInterval() method and set the time-interval to 1000ms, which is equal to one second, to call the function once per second.
- Step 8: SetInterval() will now be called first after one second of rendering, therefore call the procedure at the end to begin it exactly when the page is reloading or rendering.
Note: To make the clock look prettier, you can utilize online digital fonts. You must download their file into your project in order to use their custom font, and you must use the “font-face” property to do so.
setInterval(showTime, 1000);
function showTime() {
let time = new Date();
let hour = time.getHours();
let min = time.getMinutes();
let sec = time.getSeconds();
am_pm = "AM";
if (hour > 12) {
hour -= 12;
am_pm = "PM";
}
if (hour == 0) {
hr = 12;
am_pm = "AM";
}
hour = hour < 10 ? "0" + hour : hour;
min = min < 10 ? "0" + min : min;
sec = sec < 10 ? "0" + sec : sec;
let currentTime = hour + ":"
+ min + ":" + sec + am_pm;
document.getElementById("clock")
.innerHTML = currentTime;
}
showTime();
Another Clock Article is here 👇
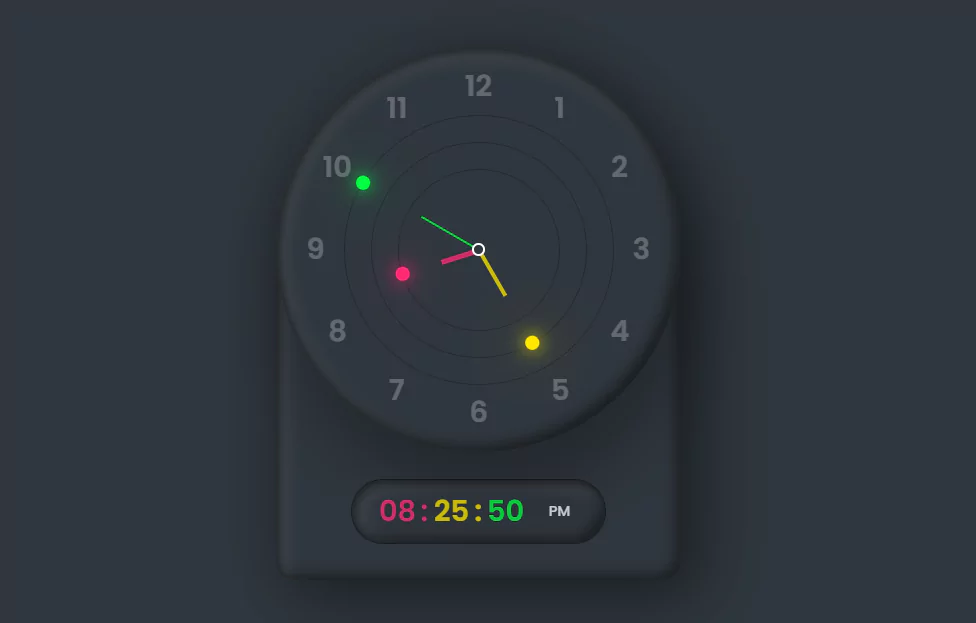