Password Validation Check in JavaScript – Show Hide Password Toggle
Today we will create a Password Validation Check in JavaScript. Also we will add Show & Hide Password Toggle functionality.
A username and password are required to log in to any website that supports authentication and authorization. If not, the user must register and generate a password in order to log in to the subsequent session, which is why websites frequently offer particular protocols or settings. When validating passwords in any format, the following parameters are frequently utilized.
- The password box only accepts alphanumeric inputs.
- Uppercase letters should be used as the first letter.
- At least one password in uppercase.
- The password needs to be a certain length.
- There can only be one digit in the password.
- There must be a minimum and maximum length for the password.
When a user creates a password, JavaScript’s password validation makes sure that these guidelines are fulfilled. The prerequisites must be satisfied in order to create a password that is at least moderately secure. JavaScript code can be used to use regular expression to validate the password’s structure.
Also, we are using HTML for the structure & CSS for Styling.
Source Code:
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Password Validation Check</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.1.2/css/all.min.css" integrity="sha512-1sCRPdkRXhBV2PBLUdRb4tMg1w2YPf37qatUFeS7zlBy7jJI8Lf4VHwWfZZfpXtYSLy85pkm9GaYVYMfw5BC1A==" crossorigin="anonymous" referrerpolicy="no-referrer" />
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="box">
<div class="inputBox">
<input type="Password" id="pswrd" placeholder="Password" onkeyup="checkPassword(this.value)">
<span id="toggleBtn"></span>
</div>
<div class="validation">
<ul>
<li id="lower">At least one lowercase character</li>
<li id="upper">At least one uppercase character</li>
<li id="number">At least one number</li>
<li id="special">At least one special character</li>
<li id="length">At least 8 characters</li>
</ul>
</div>
</div>
<script>
let pswrd = document.getElementById("pswrd");
let toggleBtn = document.getElementById("toggleBtn");
let lowerCase = document.getElementById("lower");
let upperCase = document.getElementById("upper");
let digit = document.getElementById("number");
let specialChar = document.getElementById("special");
let minLength = document.getElementById("length");
// Show hide Password
toggleBtn.onclick = function(){
if (pswrd.type === 'password') {
pswrd.setAttribute('type', 'text');
toggleBtn.classList.add('hide');
}
else {
pswrd.setAttribute('type', 'password');
toggleBtn.classList.remove('hide');
}
}
function checkPassword(data){
const lower = new RegExp('(?=.*[a-z])');
const upper = new RegExp('(?=.*[A-Z])');
const number = new RegExp('(?=.*[0-9])');
const special = new RegExp('(?=.*[!@#\$%\^&\*])');
const length = new RegExp('(?=.{8,})');
//Lower Case Validation
if(lower.test(data)){
lowerCase.classList.add('valid');
}else{
lowerCase.classList.remove('valid');
}
//Upper Case Validation
if(upper.test(data)){
upperCase.classList.add('valid');
}else{
upperCase.classList.remove('valid');
}
//Number Validation
if(number.test(data)){
digit.classList.add('valid');
}else{
digit.classList.remove('valid');
}
//Special Charater Validation
if(special.test(data)){
specialChar.classList.add('valid');
}else{
specialChar.classList.remove('valid');
}
//Password Minimum Length Validation
if(length.test(data)){
minLength.classList.add('valid');
}else{
minLength.classList.remove('valid');
}
}
</script>
</body>
</html>
Another JavaScript Project For You 👇
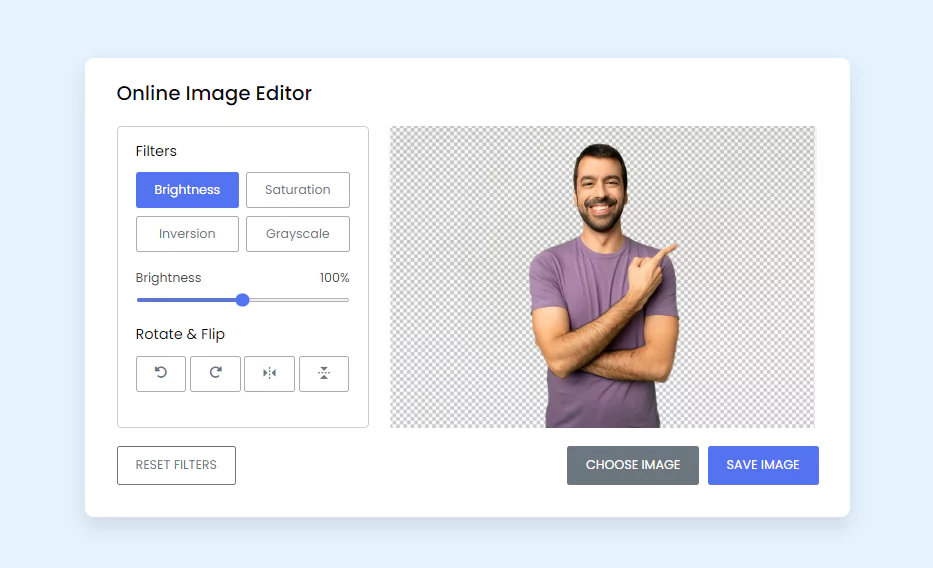
CSS:
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;500;600;700;800;900&display=swap');
*
{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body
{
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
background: #8cccff;
}
.box
{
position: relative;
width: 300px;
}
.box .inputBox
{
position: relative;
width: 100%;
background: #fff;
padding: 5px;
border-radius: 8px;
box-shadow: 0 15px 25px rgba(0,0,0,0.15);
}
.box .inputBox input
{
position: relative;
width: 100%;
outline: none;
padding: 10px 5px;
border: none;
/* border: 2px solid #999; */
}
.box .inputBox #toggleBtn
{
position: absolute;
top: 8px;
right: 10px;
width: 34px;
height: 34px;
background: rgba(0,0,0,0.05);
display: flex;
justify-content: center;
align-items: center;
cursor: pointer;
border-radius: 50%;
}
#toggleBtn::before
{
content: '\f06e';
font-family: fontAwesome;
}
#toggleBtn.hide::before
{
content: '\f070';
position: absolute;
font-family: fontAwesome;
}
.validation
{
padding: 10px;
background: #fff;
border-radius: 8px;
margin-top: 30px;
background: #376488;
box-shadow: 0 15px 25px rgba(0,0,0,0.15);
}
.validation ul
{
position: relative;
display: flex;
flex-direction: column;
gap: 8px;
}
.validation ul li
{
position: relative;
list-style: none;
color: #fff;
font-size: 0.85em;
transition: 0.5s;
}
.validation ul li.valid
{
color: rgba(255,255,255,0.5);
}
.validation ul li::before
{
content: '\f192';
font-family: fontAwesome;
width: 20px;
display: inline-flex;
}
.validation ul li.valid::before
{
content: '\f00c';
color: #0f0;
}