Text To Speech Converter Using JavaScript Project
Using JavaScript. we will build a text to speech converter application. JavaScript must be used to add a text-to-speech function to your website. The Web Speech API can be used to synthesize speech, which turns text into voice, as well as to identify speech, which turns speech into text. Yes, using JavaScript functionalities, we can create text to speech converter.
In this application, we will try to create a minimal layout. Having a space form to submit your text, then we will have a language choose option. From language option you can choose any option. Then we will have a button. So simple guys.
We will create the application structure using HTML. CSS will help to design the layout. And using JavaScript we will add all the functionalities of our application.
Source Code:
HTML:
<main>
<p class="title">Text To Speech</p>
<div class="text-section">
<p class="text-title">Enter Text</p>
<textarea name="" id="text"></textarea>
</div>
<div class="voice-section">
<p class="voice-title">Select Voice</p>
<select name="" id="voice">
<option value="Google US English">Google US English</option>
</select>
</div>
<button class="submit">Convert To Speech</button>
</main>
——————————
📂 Important Links:
——————————
>> Learn Graphics Design & Make A Successful Profession.
>> Canva Makes Graphics Design Easy.
>> Start Freelancing Today & Earn Money.
>> Make Video Editing As Your Profession.
CSS:
* {
box-sizing: border-box;
margin: 0;
padding: 0;
}
body {
background-color: rgb(94, 71, 188);
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
main {
background-color: rgb(255, 255, 255);
height: 500px;
width: 400px;
border-radius: 10px;
box-shadow: 0px 0px 60px 5px rgb(94, 71, 188);
display: flex;
justify-content: space-evenly;
align-items: center;
flex-direction: column;
}
.title {
font-size: 2rem;
font-family: Arial, Helvetica, sans-serif;
font-weight: bold;
color: rgb(48, 48, 48);
}
.text-section {
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
gap: 5px;
}
.text-title {
font-family: Arial, Helvetica, sans-serif;
font-weight: bold;
color: rgb(36, 36, 36);
align-self: start;
}
#text {
height: 7rem;
width: 20rem;
border: none;
outline: 2px solid rgba(128, 128, 128, 0.623);
border-radius: 0.5rem;
resize: none;
font-size: 15px;
font-family: Arial, Helvetica, sans-serif;
padding: 8px 10px;
}
.voice-section {
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
gap: 5px;
}
.voice-title {
font-family: Arial, Helvetica, sans-serif;
font-weight: bold;
color: rgb(36, 36, 36);
align-self: start;
}
#voice {
height: 3rem;
width: 20rem;
border: none;
outline: 2px solid rgba(128, 128, 128, 0.623);
border-radius: 0.4rem;
padding: 0px 10px;
}
.submit {
background-color: rgba(92, 55, 225, 0.979);
color: white;
width: 20rem;
height: 3rem;
border-radius: 0.3rem;
font-size: 1.2rem;
border: none;
cursor: pointer;
}
@media (max-width: 700px) {
main {
width: 60vw;
}
}
Another Interesting JavaScript Project For You.
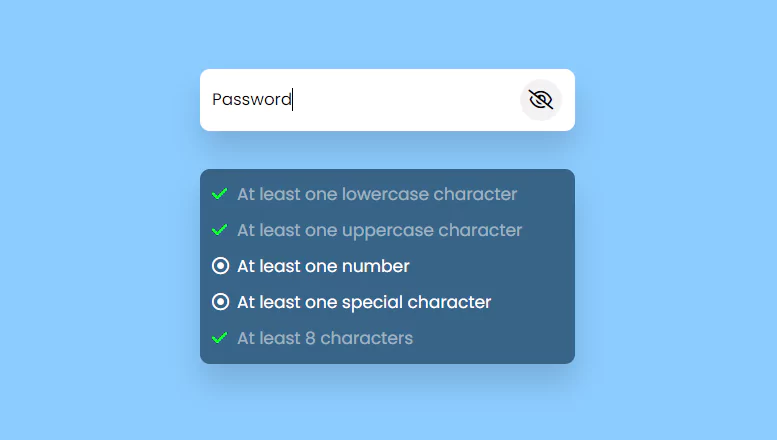
JavaScript:
const textarea = document.querySelector("#text");
let voicelist = document.querySelector("#voice");
let speechbtn = document.querySelector(".submit");
let synth = speechSynthesis;
let isSpeaking = true;
function voicespeech() {
for (let voice of synth.getVoices()) {
let option = document.createElement("option");
option.text = voice.name;
voicelist.add(option);
console.log(option);
}
}
synth.addEventListener("voiceschanged", voicespeech);
function texttospeech(text) {
let utternance = new SpeechSynthesisUtterance(text);
for (let voice of synth.getVoices()) {
if (voice.name === voicelist.value) {
utternance.voice = voice;
}
}
speechSynthesis.speak(utternance);
}
//
speechbtn.addEventListener("click", (e) => {
e.preventDefault();
if (textarea.value != "") {
if (!synth.speaking) {
texttospeech(textarea.value);
}
if (textarea.value.length > 80) {
if (isSpeaking) {
synth.resume();
isSpeaking = false;
speechbtn.innerHTML = "Pause Speech";
} else {
synth.pause();
isSpeaking = true;
speechbtn.innerHTML = "Resume Speech";
}
setInterval(() => {
if (!synth.speaking && !isSpeaking) {
isSpeaking = true;
speechbtn.innerHTML = "Convert To Speech";
}
});
} else {
speechbtn.innerHTML = "Convert To Speech";
}
}
});
voicespeech();
More Queries: