Python Project – How To Send Email Using Python
In this python project, we will create a python script for sending email. Yes, using python we can do som many interesting thing. And sending email autonomously is one of them. Hope your are all excited. Then lets do it.
Source Code
Python
import smtplib
import ssl
from email.message import EmailMessage
subject = "Email From Python"
body = "This is a test email form Python!"
sender_email = "[email protected]"
receiver_email = "[email protected]"
password = input("Enter a password: ")
message = EmailMessage()
message["From"] = sender_email
message["To"] = receiver_email
message["Subject"] = subject
html = f"""
<html>
<body>
<h1>{subject}</h1>
<p>{body}</p>
</body>
</html>
"""
message.add_alternative(html, subtype="html")
context = ssl.create_default_context()
print("Sending Email!")
with smtplib.SMTP_SSL("smtp.gmail.com", 465, context=context) as server:
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("Success")
Another Python Project For You 👇
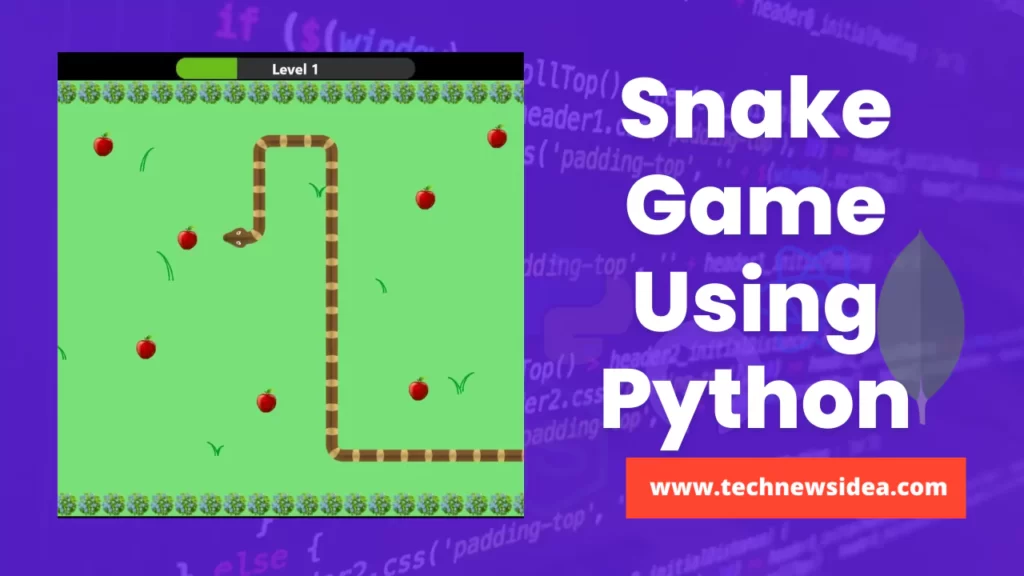