Python Project – How to Create Jarvis In Python
Hello everyone, In this python project we will build an AI Jarvis using python.
We all know what jarvis is. It is an artificial intelligence based software. Which helps to manage daily tasks easily. Today in this post, we will create a jarvis in python. We will teach our jarvis to perform some daily tasks. For doing that we will create some functions, write some code. Hopefully you will enjoy building Jarvis. Share with your friends, if you find this post helpful.
Jarvis Will Perform Certain Tasks:
- It will speak.
- Will take command.
- Wish you on your birthday.
- Will collect information from wikipedia.
- Open Daily Used Applications.
- Play your favourite song.
- You can ask the time.
- Will Open VS Code for you.
- Send email for you.
Open VS Code
In this project, I’ll be using the VS Code IDE. Use any other IDE that you are familiar with. Create a file called jarvis.py in a new project.
Define Speak Function:
def speak(audio):
pass #For now, we will write the conditions later.
Install Packages:
pip install pyttsx3
pip install pypiwin32
Define Packages:
import pyttsx3
engine = pyttsx3.init('sapi5')
voices= engine.getProperty('voices') #getting details of current voice
engine.setProperty('voice', voice[0].id)
Add speak() Function :
def speak(audio):
engine.say(audio)
engine.runAndWait() #Without this command, speech will not be audible to us.
Create main() function:
if __name__=="__main__" :
speak("Code With Harry")
Defining Wish me Function :
import datetime
def wishme():
hour = int(datetime.datetime.now().hour)
Defining Take command Function :
pip install speechRecognition
import speechRecognition as sr
def takeCommand():
#It takes microphone input from the user and returns string output
r = sr.Recognizer()
with sr.Microphone() as source:
print("Listening...")
r.pause_threshold = 1
audio = r.listen(source)
try:
print("Recognizing...")
query = r.recognize_google(audio, language='en-in') #Using google for voice recognition.
print(f"User said: {query}\n") #User query will be printed.
except Exception as e:
# print(e)
print("Say that again please...") #Say that again will be printed in case of improper voice
return "None" #None string will be returned
return query
Make Wikipedia:
pip install wikipedia
if __name__ == "__main__":
wishMe()
while True:
# if 1:
query = takeCommand().lower() #Converting user query into lower case
# Logic for executing tasks based on query
if 'wikipedia' in query: #if wikipedia found in the query then this block will be executed
speak('Searching Wikipedia...')
query = query.replace("wikipedia", "")
results = wikipedia.summary(query, sentences=2)
speak("According to Wikipedia")
print(results)
speak(results)
Open Daily Used Applications:
elif 'open youtube' in query:
webbrowser.open("youtube.com")
elif 'open google' in query:
webbrowser.open("google.com")
elif 'play music' in query:
music_dir = 'D:\\Non Critical\\songs\\Favorite Songs2'
songs = os.listdir(music_dir)
print(songs)
os.startfile(os.path.join(music_dir, songs[0]))
Ask The Time:
elif 'the time' in query:
strTime = datetime.datetime.now().strftime("%H:%M:%S")
speak(f"Sir, the time is {strTime}")
Open VS Code:
elif 'open code' in query:
codePath = "C:\Users\DELL\AppData\Local\Programs\Microsoft VS Code\Code.exe"
os.startfile(codePath)
Create sendEmail() function to send email:
def sendEmail(to, content):
server = smtplib.SMTP('smtp.gmail.com', 587)
server.ehlo()
server.starttls()
server.login('[email protected]', 'your-password')
server.sendmail('[email protected]', to, content)
server.close()
Calling sendEmail() function inside the main() function:
elif 'email to john' in query:
try:
speak("What should I say?")
content = takeCommand()
to = "[email protected]"
sendEmail(to, content)
speak("Email has been sent!")
except Exception as e:
print(e)
speak("Sorry Boss. I am not able to send this email")
Another Python Project For You 👇
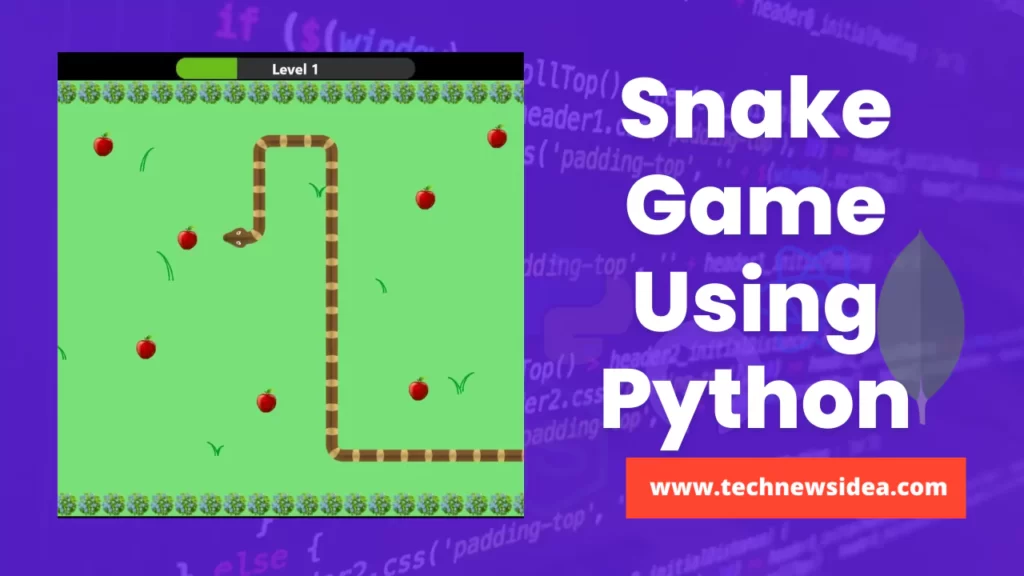
Complete Jarvis Code:
import pyttsx3 #pip install pyttsx3
import speech_recognition as sr #pip install speechRecognition
import datetime
import wikipedia #pip install wikipedia
import webbrowser
import os
import smtplib
engine = pyttsx3.init('sapi5')
voices = engine.getProperty('voices')
# print(voices[1].id)
engine.setProperty('voice', voices[0].id)
def speak(audio):
engine.say(audio)
engine.runAndWait()
def wishMe():
hour = int(datetime.datetime.now().hour)
if hour>=0 and hour<12:
speak("Good Morning!")
elif hour>=12 and hour<18:
speak("Good Afternoon!")
else:
speak("Good Evening!")
speak("I am Jarvis Sir. Please tell me how may I help you")
def takeCommand():
#It takes microphone input from the user and returns string output
r = sr.Recognizer()
with sr.Microphone() as source:
print("Listening...")
r.pause_threshold = 1
audio = r.listen(source)
try:
print("Recognizing...")
query = r.recognize_google(audio, language='en-in')
print(f"User said: {query}\n")
except Exception as e:
# print(e)
print("Say that again please...")
return "None"
return query
def sendEmail(to, content):
server = smtplib.SMTP('smtp.gmail.com', 587)
server.ehlo()
server.starttls()
server.login('[email protected]', 'your-password')
server.sendmail('[email protected]', to, content)
server.close()
if __name__ == "__main__":
wishMe()
while True:
# if 1:
query = takeCommand().lower()
# Logic for executing tasks based on query
if 'wikipedia' in query:
speak('Searching Wikipedia...')
query = query.replace("wikipedia", "")
results = wikipedia.summary(query, sentences=2)
speak("According to Wikipedia")
print(results)
speak(results)
elif 'open youtube' in query:
webbrowser.open("youtube.com")
elif 'open google' in query:
webbrowser.open("google.com")
elif 'open stackoverflow' in query:
webbrowser.open("stackoverflow.com")
elif 'play music' in query:
music_dir = 'D:\\Non Critical\\songs\\Favorite Songs2'
songs = os.listdir(music_dir)
print(songs)
os.startfile(os.path.join(music_dir, songs[0]))
elif 'the time' in query:
strTime = datetime.datetime.now().strftime("%H:%M:%S")
speak(f"Sir, the time is {strTime}")
elif 'open code' in query:
codePath = "C:\Users\DELL\AppData\Local\Programs\Microsoft VS Code\Code.exe"
os.startfile(codePath)
elif 'email to john' in query:
try:
speak("What should I say?")
content = takeCommand()
to = "[email protected]"
sendEmail(to, content)
speak("Email has been sent!")
except Exception as e:
print(e)
speak("Sorry Boss. I am not able to send this email")
jarvis ai python code download
how to make your own ai assistant in python