Create a Review carousel – JavaScript Project
You will learn how to make a carousel of reviews using a button that creates random reviews in this lesson. We will use HTML, CSS & JavaScript to create this project.
This is a useful tool to have for displaying client reviews on a personal portfolio or an e-commerce website.
JavaScript Fundamentals will be covered in this project:
- objects
- DOMContentLoaded
- addEventListener()
- array.length
- textContent
Source Code:
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Starter</title>
<!-- font-awesome -->
<link
rel="stylesheet"
href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.14.0/css/all.min.css"
/>
<!-- styles -->
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<main>
<section class="container">
<!-- title -->
<div class="title">
<h2>our reviews</h2>
<div class="underline"></div>
</div>
<!-- review -->
<article class="review">
<div class="img-container">
<img src="person-1.jpeg" id="person-img" alt="" />
</div>
<h4 id="author">sara jones</h4>
<p id="job">ux designer</p>
<p id="info">
Lorem ipsum dolor sit amet consectetur adipisicing elit. Iusto
asperiores debitis incidunt, eius earum ipsam cupiditate libero?
Iste, doloremque nihil?
</p>
<!-- prev next buttons-->
<div class="button-container">
<button class="prev-btn">
<i class="fas fa-chevron-left"></i>
</button>
<button class="next-btn">
<i class="fas fa-chevron-right"></i>
</button>
</div>
<!-- random button -->
<button class="random-btn">surprise me</button>
</article>
</section>
</main>
<!-- javascript -->
<script src="app.js"></script>
</body>
</html>
Another JavaScript Project For You.
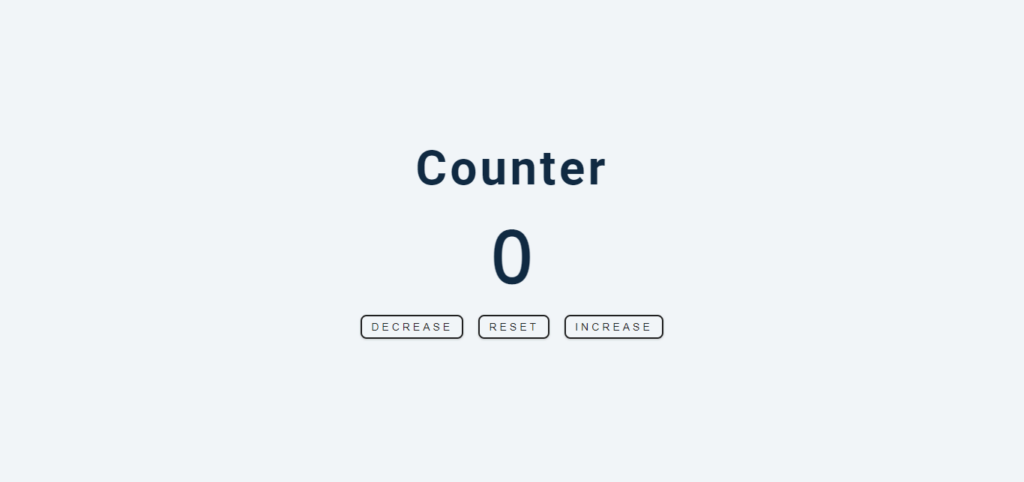
CSS:
/*
===============
Fonts
===============
*/
@import url("https://fonts.googleapis.com/css?family=Open+Sans|Roboto:400,700&display=swap");
/*
===============
Variables
===============
*/
:root {
/* dark shades of primary color*/
--clr-primary-1: hsl(205, 86%, 17%);
--clr-primary-2: hsl(205, 77%, 27%);
--clr-primary-3: hsl(205, 72%, 37%);
--clr-primary-4: hsl(205, 63%, 48%);
/* primary/main color */
--clr-primary-5: hsl(205, 78%, 60%);
/* lighter shades of primary color */
--clr-primary-6: hsl(205, 89%, 70%);
--clr-primary-7: hsl(205, 90%, 76%);
--clr-primary-8: hsl(205, 86%, 81%);
--clr-primary-9: hsl(205, 90%, 88%);
--clr-primary-10: hsl(205, 100%, 96%);
/* darkest grey - used for headings */
--clr-grey-1: hsl(209, 61%, 16%);
--clr-grey-2: hsl(211, 39%, 23%);
--clr-grey-3: hsl(209, 34%, 30%);
--clr-grey-4: hsl(209, 28%, 39%);
/* grey used for paragraphs */
--clr-grey-5: hsl(210, 22%, 49%);
--clr-grey-6: hsl(209, 23%, 60%);
--clr-grey-7: hsl(211, 27%, 70%);
--clr-grey-8: hsl(210, 31%, 80%);
--clr-grey-9: hsl(212, 33%, 89%);
--clr-grey-10: hsl(210, 36%, 96%);
--clr-white: #fff;
--clr-red-dark: hsl(360, 67%, 44%);
--clr-red-light: hsl(360, 71%, 66%);
--clr-green-dark: hsl(125, 67%, 44%);
--clr-green-light: hsl(125, 71%, 66%);
--clr-black: #222;
--ff-primary: "Roboto", sans-serif;
--ff-secondary: "Open Sans", sans-serif;
--transition: all 0.3s linear;
--spacing: 0.1rem;
--radius: 0.25rem;
--light-shadow: 0 5px 15px rgba(0, 0, 0, 0.1);
--dark-shadow: 0 5px 15px rgba(0, 0, 0, 0.2);
--max-width: 1170px;
--fixed-width: 620px;
}
/*
===============
Global Styles
===============
*/
*,
::after,
::before {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: var(--ff-secondary);
background: var(--clr-grey-10);
color: var(--clr-grey-1);
line-height: 1.5;
font-size: 0.875rem;
}
ul {
list-style-type: none;
}
a {
text-decoration: none;
}
h1,
h2,
h3,
h4 {
letter-spacing: var(--spacing);
text-transform: capitalize;
line-height: 1.25;
margin-bottom: 0.75rem;
font-family: var(--ff-primary);
}
h1 {
font-size: 3rem;
}
h2 {
font-size: 2rem;
}
h3 {
font-size: 1.25rem;
}
h4 {
font-size: 0.875rem;
}
p {
margin-bottom: 1.25rem;
color: var(--clr-grey-5);
}
@media screen and (min-width: 800px) {
h1 {
font-size: 4rem;
}
h2 {
font-size: 2.5rem;
}
h3 {
font-size: 1.75rem;
}
h4 {
font-size: 1rem;
}
body {
font-size: 1rem;
}
h1,
h2,
h3,
h4 {
line-height: 1;
}
}
/* global classes */
/* section */
.section {
padding: 5rem 0;
}
.section-center {
width: 90vw;
margin: 0 auto;
max-width: 1170px;
}
@media screen and (min-width: 992px) {
.section-center {
width: 95vw;
}
}
main {
min-height: 100vh;
display: grid;
place-items: center;
}
/*
===============
Reviews
===============
*/
main {
min-height: 100vh;
display: grid;
place-items: center;
}
.title {
text-align: center;
margin-bottom: 4rem;
}
.underline {
height: 0.25rem;
width: 5rem;
background: var(--clr-primary-5);
margin-left: auto;
margin-right: auto;
}
.container {
width: 80vw;
max-width: var(--fixed-width);
}
.review {
background: var(--clr-white);
padding: 1.5rem 2rem;
border-radius: var(--radius);
box-shadow: var(--light-shadow);
transition: var(--transition);
text-align: center;
}
.review:hover {
box-shadow: var(--dark-shadow);
}
.img-container {
position: relative;
width: 150px;
height: 150px;
border-radius: 50%;
margin: 0 auto;
margin-bottom: 1.5rem;
}
#person-img {
width: 100%;
display: block;
height: 100%;
object-fit: cover;
border-radius: 50%;
position: relative;
}
.img-container::after {
font-family: "Font Awesome 5 Free";
font-weight: 900;
content: "\f10e";
position: absolute;
top: 0;
left: 0;
width: 2.5rem;
height: 2.5rem;
display: grid;
place-items: center;
border-radius: 50%;
transform: translateY(25%);
background: var(--clr-primary-5);
color: var(--clr-white);
}
.img-container::before {
content: "";
width: 100%;
height: 100%;
background: var(--clr-primary-5);
position: absolute;
top: -0.25rem;
right: -0.5rem;
border-radius: 50%;
}
#author {
margin-bottom: 0.25rem;
}
#job {
margin-bottom: 0.5rem;
text-transform: uppercase;
color: var(--clr-primary-5);
font-size: 0.85rem;
}
#info {
margin-bottom: 0.75rem;
}
.prev-btn,
.next-btn {
color: var(--clr-primary-7);
font-size: 1.25rem;
background: transparent;
border-color: transparent;
margin: 0 0.5rem;
transition: var(--transition);
cursor: pointer;
}
.prev-btn:hover,
.next-btn:hover {
color: var(--clr-primary-5);
}
.random-btn {
margin-top: 0.5rem;
background: var(--clr-primary-10);
color: var(--clr-primary-5);
padding: 0.25rem 0.5rem;
text-transform: capitalize;
border-radius: var(--radius);
transition: var(--transition);
border-color: var(--clr-primary-5);
cursor: pointer;
}
.random-btn:hover {
background: var(--clr-primary-5);
color: var(--clr-primary-1);
}
We know, you can copy the code from here. But if you want to support us, then you can buy the code from here. It will help us to share more free resources.
JavaScript:
// local reviews data
const reviews = [
{
id: 1,
name: "susan smith",
job: "web developer",
img:
"https://res.cloudinary.com/diqqf3eq2/image/upload/v1586883334/person-1_rfzshl.jpg",
text:
"I'm baby meggings twee health goth +1. Bicycle rights tumeric chartreuse before they sold out chambray pop-up. Shaman humblebrag pickled coloring book salvia hoodie, cold-pressed four dollar toast everyday carry",
},
{
id: 2,
name: "anna johnson",
job: "web designer",
img:
"https://res.cloudinary.com/diqqf3eq2/image/upload/v1586883409/person-2_np9x5l.jpg",
text:
"Helvetica artisan kinfolk thundercats lumbersexual blue bottle. Disrupt glossier gastropub deep v vice franzen hell of brooklyn twee enamel pin fashion axe.photo booth jean shorts artisan narwhal.",
},
{
id: 3,
name: "peter jones",
job: "intern",
img:
"https://res.cloudinary.com/diqqf3eq2/image/upload/v1586883417/person-3_ipa0mj.jpg",
text:
"Sriracha literally flexitarian irony, vape marfa unicorn. Glossier tattooed 8-bit, fixie waistcoat offal activated charcoal slow-carb marfa hell of pabst raclette post-ironic jianbing swag.",
},
{
id: 4,
name: "bill anderson",
job: "the boss",
img:
"https://res.cloudinary.com/diqqf3eq2/image/upload/v1586883423/person-4_t9nxjt.jpg",
text:
"Edison bulb put a bird on it humblebrag, marfa pok pok heirloom fashion axe cray stumptown venmo actually seitan. VHS farm-to-table schlitz, edison bulb pop-up 3 wolf moon tote bag street art shabby chic. ",
},
];
// select items
const img = document.getElementById("person-img");
const author = document.getElementById("author");
const job = document.getElementById("job");
const info = document.getElementById("info");
const prevBtn = document.querySelector(".prev-btn");
const nextBtn = document.querySelector(".next-btn");
const randomBtn = document.querySelector(".random-btn");
// set starting item
let currentItem = 0;
// load initial item
window.addEventListener("DOMContentLoaded", function () {
const item = reviews[currentItem];
img.src = item.img;
author.textContent = item.name;
job.textContent = item.job;
info.textContent = item.text;
});
// show person based on item
function showPerson(person) {
const item = reviews[person];
img.src = item.img;
author.textContent = item.name;
job.textContent = item.job;
info.textContent = item.text;
}
// show next person
nextBtn.addEventListener("click", function () {
currentItem++;
if (currentItem > reviews.length - 1) {
currentItem = 0;
}
showPerson(currentItem);
});
// show prev person
prevBtn.addEventListener("click", function () {
currentItem--;
if (currentItem < 0) {
currentItem = reviews.length - 1;
}
showPerson(currentItem);
});
// show random person
randomBtn.addEventListener("click", function () {
console.log("hello");
currentItem = Math.floor(Math.random() * reviews.length);
showPerson(currentItem);
});