GSAP Image Slider Animation With HTML CSS JavaScript
Using HTML, CSS, and JavaScript GSAP, we will create an animated image slider. In this tutorial, I’ll show you how to make an animated image slider using HTML, CSS, and JavaScript.
The structure will be created using HTML. The styling will be added using CSS, and JavaScript will be used for specific features. For additional HTML, CSS, and JavaScript projects, see our website.
Source Code:
HTML:
<!DOCTYPE html>
<html lang="en" >
<head>
<meta charset="UTF-8">
<title>Elastic Accordion GSAP</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/meyer-reset/2.0/reset.min.css">
<link rel="stylesheet" href="./style.css">
</head>
<body>
<!-- partial:index.partial.html -->
<div class="group">
<div class="item" style="background-image: url(https://c4.wallpaperflare.com/wallpaper/815/711/721/anime-boy-fight-knife-wallpaper-preview.jpg)"></div>
<div class="item" style="background-image: url(https://w0.peakpx.com/wallpaper/913/450/HD-wallpaper-senjin-female-dress-sexy-warrior-girl-blade-emotional-anime-hot-petals-anime-girl-long-hair-sword-serious.jpg)"></div>
<div class="item" style="background-image: url(https://e0.pxfuel.com/wallpapers/182/524/desktop-wallpaper-game-application-digital-art-women-samurai-warrior-anime-samurai-art.jpg)"></div>
<div class="item" style="background-image: url(https://rare-gallery.com/thumbs/4512738-black-eyes-guweiz-short-hair-armor-black-hair-gloves-headdress-japanese-clothes-rain-samurai-umbrella-water.jpg)"></div>
<div class="item" style="background-image: url(https://i.pinimg.com/originals/d8/ef/da/d8efda191c6736020d29fd4780204e15.jpg)"></div>
</div>
<!-- partial -->
<script src='https://unpkg.co/gsap@3/dist/gsap.min.js'></script><script src="./script.js"></script>
</body>
</html>
——————————
📂 Important Links:
——————————
>> Learn Graphics Design & Make A Successful Profession.
>> Canva Makes Graphics Design Easy.
>> Start Freelancing Today & Earn Money.
>> Make Video Editing As Your Profession.
CSS:
body {
height: 100vh;
display: flex;
margin: 0;
background: linear-gradient(135deg, #f53844, #fc9842);
align-items: center;
justify-content: center;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
.group {
text-align: center;
white-space: nowrap;
overflow: hidden;
}
.item {
width: 12vw;
height: 75vh;
background-position: top;
background-size: cover 75vh;
margin: 0.7vw;
border-radius: 2.5vw;
display: inline-block;
cursor: pointer;
box-shadow: 1px 3px 10px rgba(0, 0, 0, 0.5);
}
ANOTHER article for you.
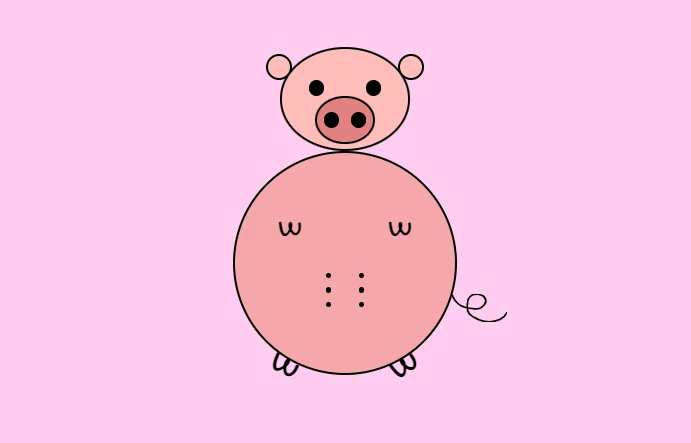
JavaScript:
const items = document.querySelectorAll(".item");
const expand = (item, i) => {
items.forEach((it, ind) => {
if (i === ind) return;
it.clicked = false;
});
gsap.to(items, {
width: item.clicked ? "15vw" : "8vw",
duration: 2,
ease: "elastic(1, .6)" });
item.clicked = !item.clicked;
gsap.to(item, {
width: item.clicked ? "42vw" : "15vw",
duration: 2.5,
ease: "elastic(1, .3)" });
};
items.forEach((item, i) => {
item.clicked = false;
item.addEventListener("click", () => expand(item, i));
});